Quick integration
Create an application
- To log in, visit the website and click on the "login" button located in the top right corner.
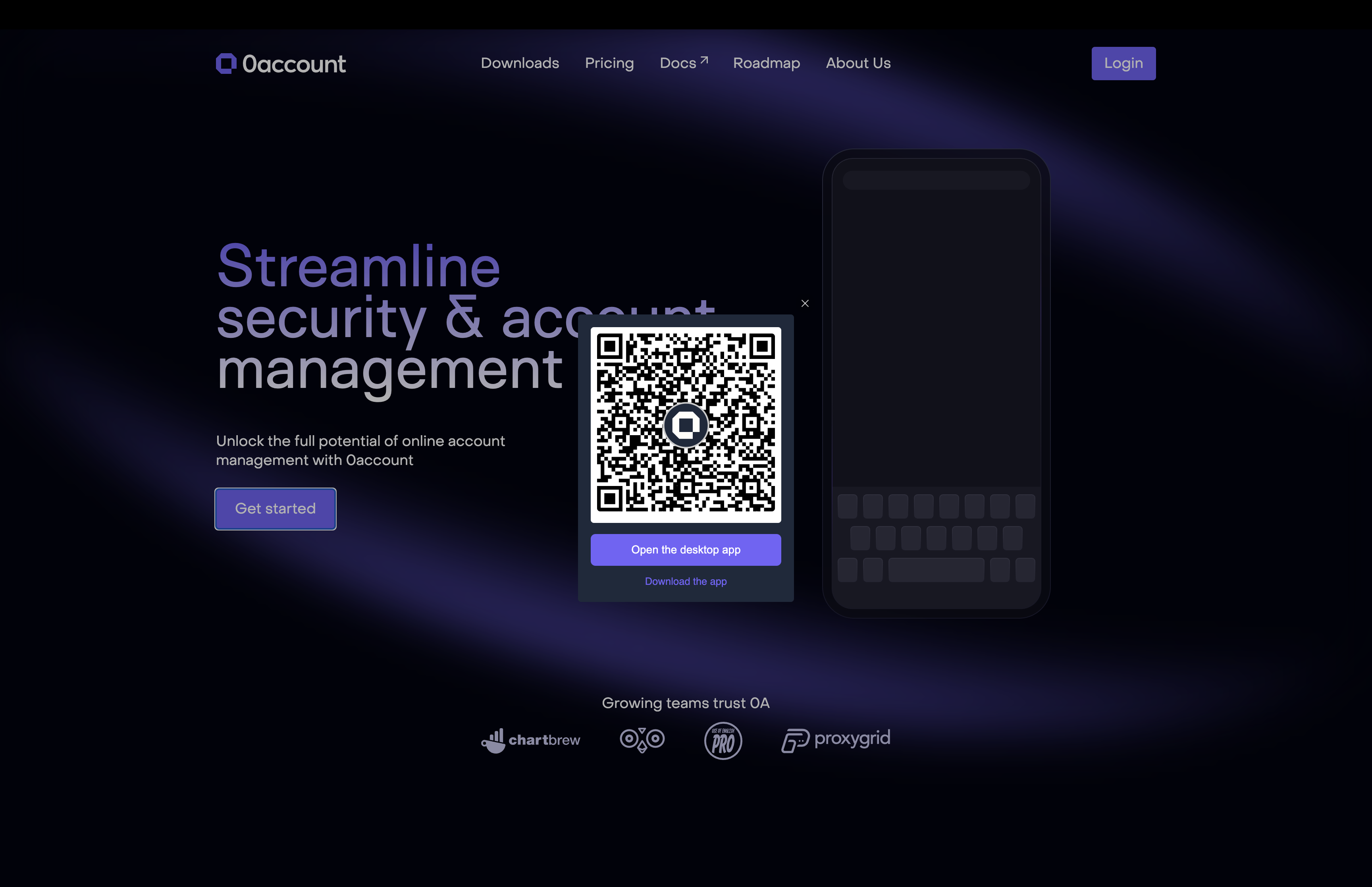
-
Download 0account app if you haven’t already.
-
Authenticate on the website using the app.
tip
In case you want to authenticate using mobile app, you can scan the QR code.
- Create a new app:
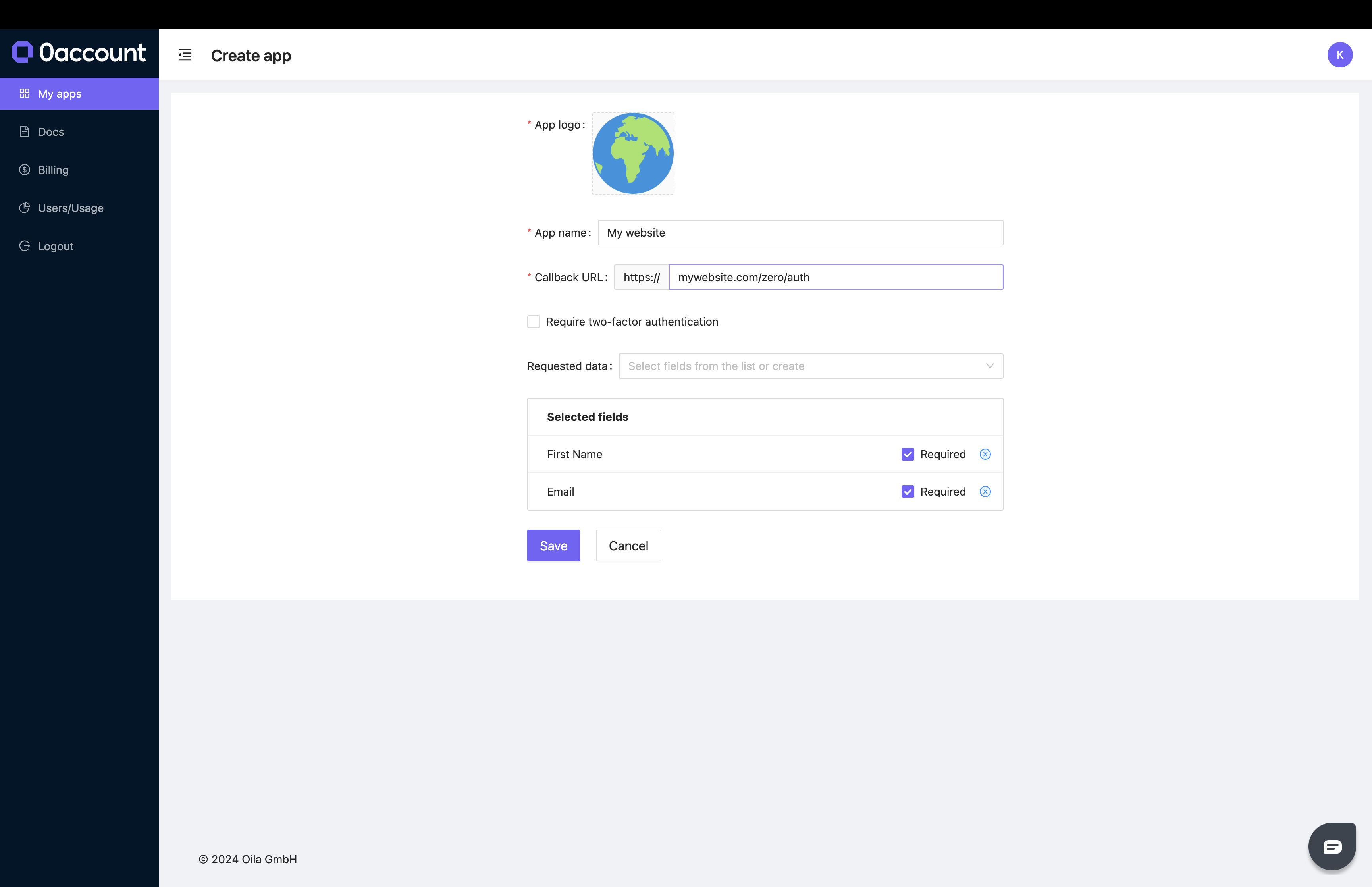
- Copy the app ID to be used for frontend integration.
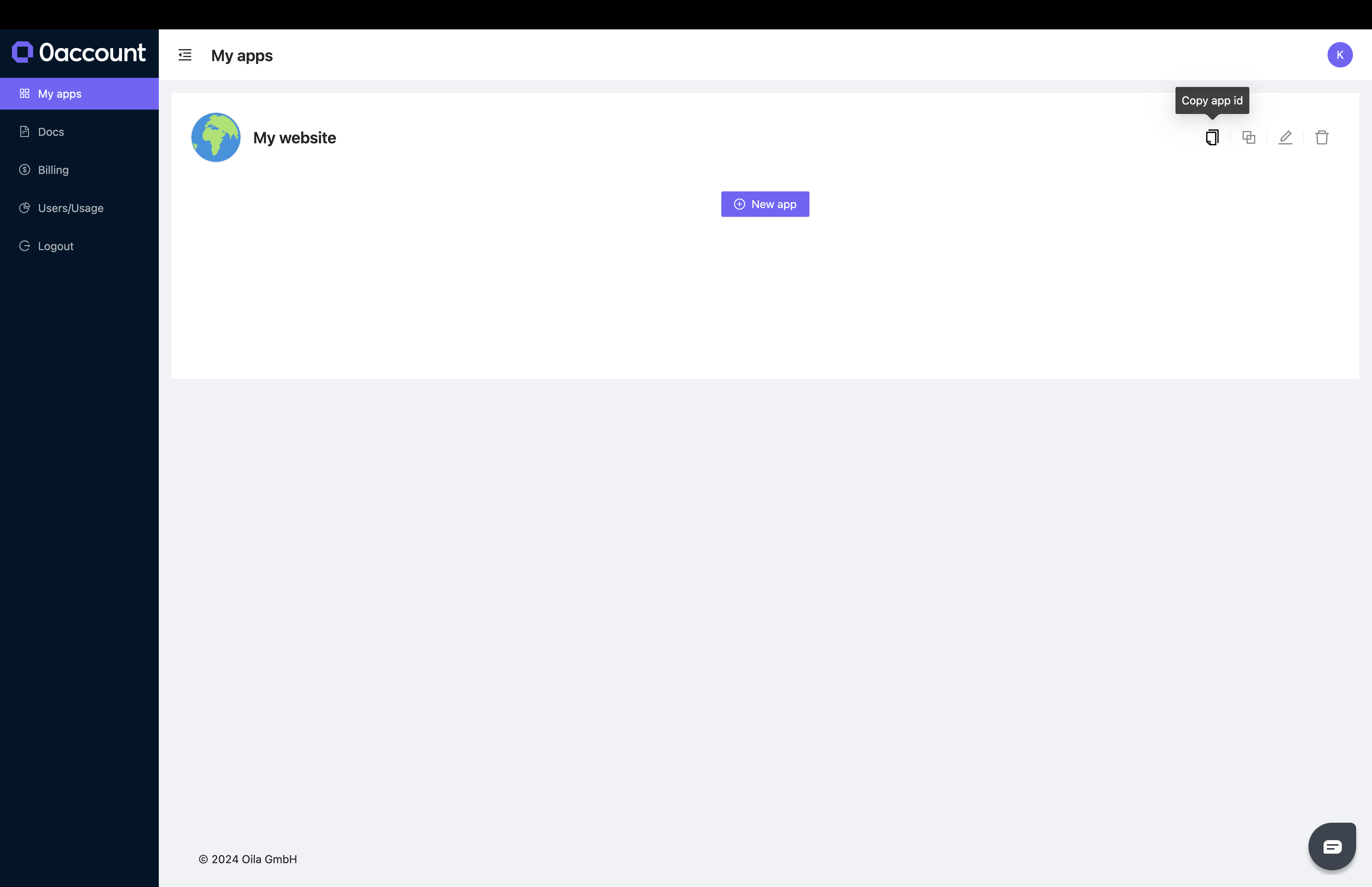
- Copy the app secret to be used for backend integration.
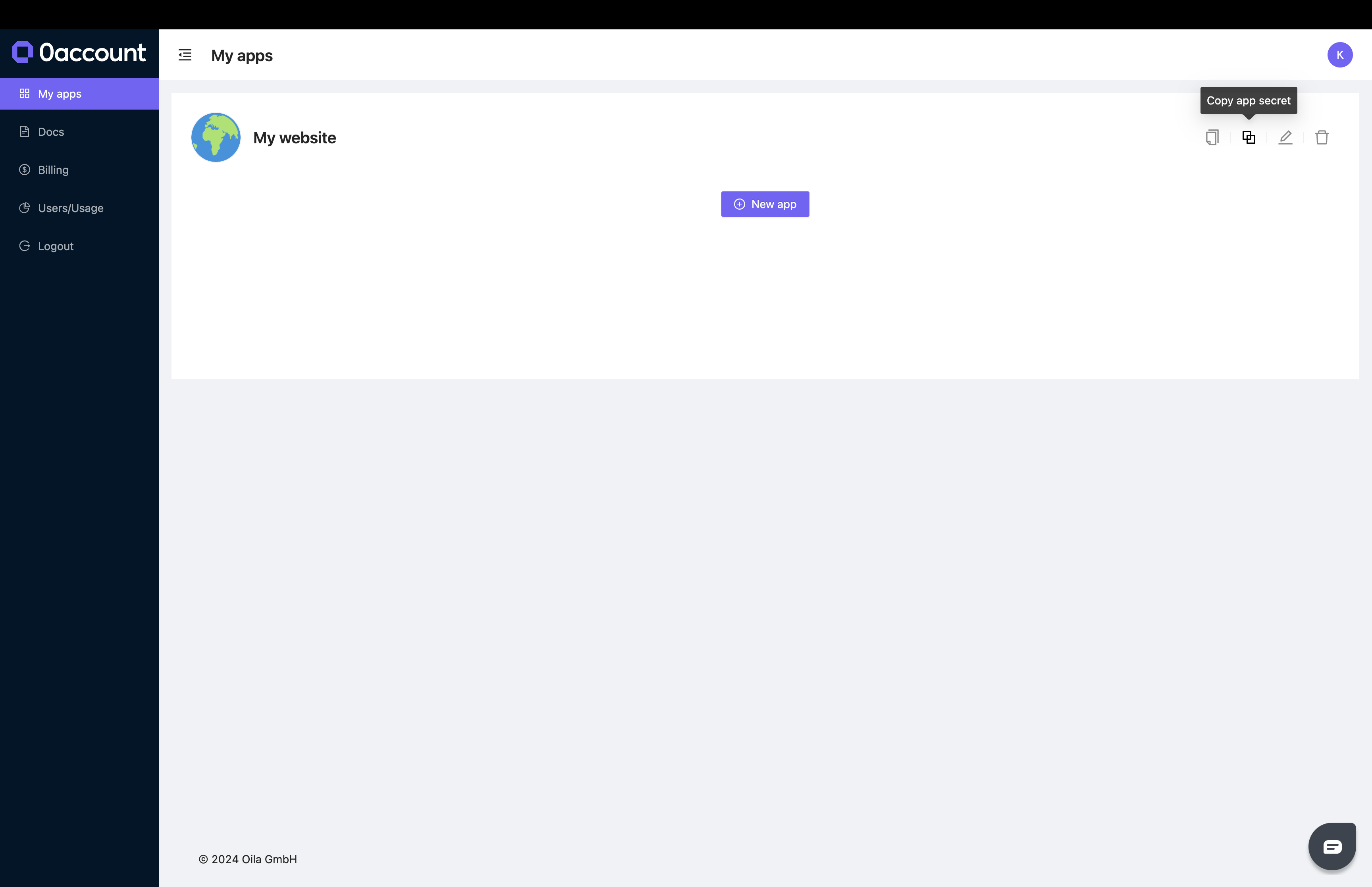
Now we can move on and implement frontend related part.
Frontend
- npm
- Browser (CDN)
npm install @oila/0account
<script type="module" src="https://cdn.jsdelivr.net/npm/@oila/[email protected]/dist/zero-account/zero-account.esm.js"></script>
Import in the library SDK:
- Web component
- Angular
<zero-account app-id="{YOUR_APP_ID}" theme-preset='dark' />
Coming soon, please use web component as for now
<zero-account app-id="{YOUR_APP_ID}" theme-preset='dark' />
tip
For a complete list of customization options, please visit the customizations page.
Handle authentication
To complete the authentication process, you need to listen for the "0account-authenticated" event.
document.addEventListener("0account-authenticated", (event) => {
// data contains any data you have returned from the backend
const data = event.detail;
// your business logic here. This is a good place to redirect the user.
});
If you want to handle remote logout from 0account app, handle the "0account-logout" event.
document.addEventListener("0account-logout", (_) => {
// implement your logout logic here.
});
Frontend is done and let's get to the fun part.
Backend
- Golang
- Node.js
- Python
- PHP
- WordPress
- iOS
- Android
go get "github.com/oila-gmbh/0account-go"
npm install 0account-node
pip install 0account-python
Coming soon
Coming soon
Coming soon
Coming soon
Connect 0account middleware
- Golang
- Node.js
- Python
- PHP
- WordPress
- iOS
- Android
package main
import (
"encoding/json"
"net/http"
"os"
"github.com/go-redis/redis/v9"
zero "github.com/oila-gmbh/0account-go"
)
func main() {
var redisClient = redis.NewClient(&redis.Options{})
// This is the secret from 0account app, we created earlier
zero.SetAppSecret(os.Getenv("ZERO_APP_SECRET"))
// If engine is not provided, an in-memory engine will be used.
// For production, it is recommended to provide an engine:
// for this example we will use redis but any other database could be used
zero.SetEngineSetterAndGetter(
func(ctx context.Context, k string, v []byte) error {
// For the best results the timeout should match the timeout
// set in frontend (updateInterval option, default: 3 minutes)
return redisClient.Set(ctx, k, v, 3*time.Minute)
},
func(ctx context.Context, k string) ([]byte, error) {
return redisClient.Get(ctx, k)
},
)
// The route URL is the callback URL you have set when you created 0account app.
http.Handle("/zero/auth", func(w http.ResponseWriter, r *http.Request) {
// We can get any data we defined in the requested data section in the admin panel
// NOTE: all json data should be camel-cased, e.g. Last Name should be lastName
type User struct {
FirstName string `json:"firstName"`
}
user, metadata, err := zero.Auth[User](context.Background(), r.Header, r.Body))
if err != nil {
http.Error(w, "User is not authorized", http.StatusUnauthorized)
return
}
// If the request comes from 0account webhook, we must return success,
// so it knows that the library saved the data
if metadata.IsWebhookRequest() {
w.WriteHeader(http.StatusOK)
return
}
// any data returned here would be sent to 0account-authenticated event on front-end e.g.:
w.Header().Set("Content-Type", "application/json")
w.WriteHeader(http.StatusOK)
userData := map[string]string{"firstName": user.FirstName}
json.NewEncoder(w).Encode(userData)
})
}
const express = require('express');
const { ZeroAccount } = require('0account-node');
const Redis = require('ioredis');
const app = express();
const redis = new Redis();
app.use(express.json());
const redisEngine = {
// For the best results the timeout should match the timeout
// set in frontend (updateInterval option, default: 3 minutes)
set: (k,v) => redis.set(k, v, "EX", 3 * 60),
get: (k) => redis.get(k)
};
const zero = new ZeroAccount(
// This is the secret from 0account app, we created earlier
process.env.ZERO_APP_SECRET,
// If engine is not provided, an in-memory engine will be used.
// For production, it is recommended to provide an engine:
// for this example we will use redis but any other database could be used
redisEngine
)
// The route URL is the callback URL you have set when you created 0account app.
app.post('/zero/auth', async (req, res) => {
try {
const { data, metadata } = await zero.auth(req.headers, req.body)
// If the request comes from 0account webhook, we must return success,
// so it knows that the library saved the data
if (metadata.isWebhookRequest) return res.sendStatus(200);
// We can get any data we defined in the requested data section in the admin panel
// NOTE: all json data should be camel-cased, e.g. Last Name should be lastName
const { firstName } = data;
// Any data returned here would be sent to 0account-authenticated event on front-end e.g.:
return res.status(200).json({ firstName });
} catch (err) {
return res.status(401).json({ error: 'User is not authorized' })
}
})
app.listen(process.env.PORT || 3000);
from fastapi import FastAPI, Request, Response, status
from pydantic import BaseModel
import redis
from zeroaccount import ZeroAccount
app = FastAPI()
redis_client = redis.Redis()
class RedisEngine:
def set(self, key: str, value: str) -> None:
# For the best results the timeout should match the timeout
# set in frontend (updateInterval option, default: 3 minutes)
redis_client.set(key, value, 3 * 60)
def get(self, key: str) -> str:
return redis_client.get(key)
zero = ZeroAccount(
# This is the secret from 0account app, we created earlier
os.environ.get('ZERO_APP_SECRET'),
# If engine is not provided, an in-memory engine will be used.
# For production, it is recommended to provide an engine:
# for this example we will use redis but any other database could be used
new RedisEngine(),
)
# The route URL is the callback URL you have set when you created 0account app.
@app.post("/zero/auth", status_code=200)
async def zero_auth(request: Request, response: Response):
try:
result = zero.auth(request.headers, await request.json())
data = result.data
meta = result.metadata
# If the request comes from 0account webhook, we must return success,
# so it knows that the library saved the data
if meta.is_webhook_request():
response.status_code = status.HTTP_200_OK
return
# We can get any data we defined in the requested data section in the admin panel
# NOTE: all json data should be camel-cased, e.g. Last Name should be lastName
first_name = data.get('firstName', 'Anonymous')
# Any data returned here would be sent to 0account-authenticated event on front-end e.g.:
return {"firstName": first_name}
except:
raise HTTPException(status_code=401, detail="User is not authorized")
<?php
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Route;
use Illuminate\Support\Facades\Redis;
use Oila\ZeroAccount\ZeroAccount;
use Oila\ZeroAccount\Engine;
final class RedisEngine implements Engine
{
public function set(string $key, array $value): void
{
// For the best results the timeout should match the timeout
// set in frontend (updateInterval option, default: 3 minutes)
Redis::set($key, json_encode($value), 'EX', 3 * 60);
}
public function get(string $key): array
{
return json_decode(Redis::get($key), true);
}
}
global $zero;
$zero = new ZeroAccount(
// This is the secret from 0account app, we created earlier
getenv('ZERO_APP_SECRET'),
// If engine is not provided, an in-memory engine will be used.
// For production, it is recommended to provide an engine:
// for this example we will use redis but any other database could be used
new RedisEngine(),
);
// The route URL is the callback URL you have set when you created 0account app.
Route::post('/zero/auth', function (Request $request) {
global $zero;
try {
$result = $zero->auth($request->header(), $request->getContent());
// If the request comes from 0account webhook, we must return success,
// so it knows that the library saved the data
if ($result->metadata()->isWebhookRequest()) {
return response()->noContent(200);
}
$data = $result->data();
// We can get any data we defined in the requested data section in the admin panel
// NOTE: all json data should be camel-cased, e.g. Last Name should be lastName
$firstName = $data['firstName'];
// Any data returned here would be sent to 0account-authenticated event on front-end e.g.:
return response()->json(['firstName' => $firstName]);
} catch (Exception $e) {
// handle exception
return response()->json([
'message' => 'User is not authorized'
], 401);
}
});
Coming soon
Coming soon
Coming soon